These problems can be a real pain. However, they’re avoidable with proper email validation and verification. There are plenty of ways to do this and validating them with PHP is one of the best out there.
In this guide, we’ll walk you through PHP techniques and tips to help you implement robust email verification and validation strategies.
Let’s dive into how you can use PHP to prevent email headaches!
Email validation and verification in PHP
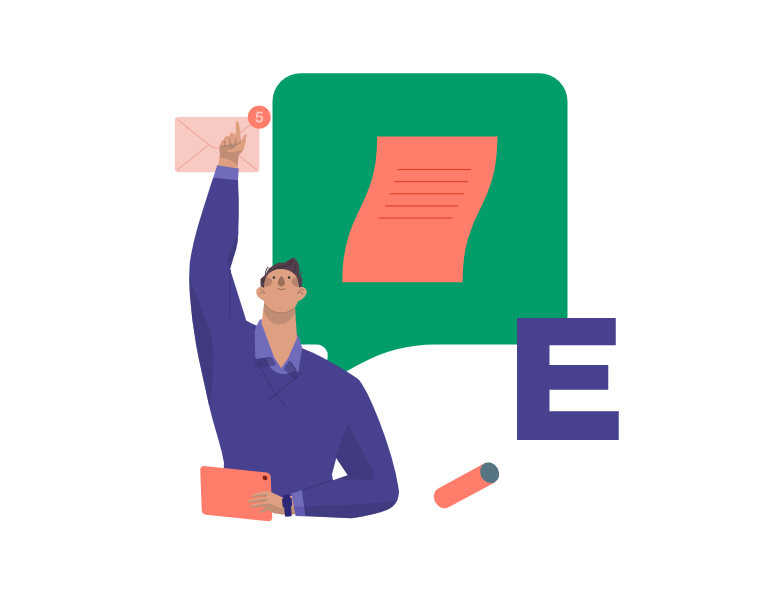
Email validation and email verification are two fundamental processes.
The first focuses on checking if the email’s syntax conforms to established standards, while the second goes a step further and verifies that the address exists and can receive messages.
These processes can show whether you are dealing with valid email addresses.
Even better, they can reduce bounce rates, prevent spam, and keep your communications flowing.
PHP language is a powerful tool in this area.
With its built-in functions, such as filter_var() and getmxrr(), PHP provides an easy way to incorporate email verification and validation processes without requiring external libraries or APIs.
Beyond that, using PHP for validation helps maintain clean data, so your marketing campaigns and newsletters hit real inboxes rather than fake or abandoned accounts.
Importance of accurate email validation checks
When users sign up for a service or submit forms, they might accidentally input an incorrect or invalid email address. For instance, typos in the domain part (e.g., “user@gnail.com” instead of “user@gmail.com”) can easily slip by.
Checking the accuracy of email validation helps avoid bounces and streamlines your communication.
It will also reduce spam.
Spam, or unsolicited emails, can clog up inboxes and waste valuable time. When you validate email addresses and get accurate results, you can filter out invalid addresses used by spammers.
Accurate checks also help prevent fake sign-ups.
Bots often fill out forms with illegal characters or random Unicode characters. If not caught early, you might end up with a database full of unusable contacts.
Email validation vs. email verification
Email validation is typically used to confirm if an email address is well-formed and adheres to the standard email syntax.
It can handle basic Latin script characters, but it may not validate addresses that contain international characters correctly.
Using PHP’s built-in filter_var() function, you can analyze an email’s structure to see if it meets the standard requirements and even IP addresses to ensure they are accessible.
For example:
However, validation only ensures that the email address is syntactically correct. It doesn’t tell you whether the email exists.
Email verification, on the other hand, can prove that the email address is valid in structure, exists, belongs to an actual user, and can receive messages.
One way to achieve this is by checking the MX records for the domain part of the email. The MX record tells you if a mail server is configured to handle emails for that domain.
Here’s how you can do that in PHP:
In the above example, PHP’s checkdnsrr() function examines if the valid domain name has a corresponding MX record. If it does, that means the domain can accept emails.
When to use validation and when verification
Validation is often enough when collecting emails for less critical interactions, such as user registrations, newsletters, or contact forms.
Verification is used to handle more sensitive or essential communications. For instance, you might send a verification email to the address and ask the recipient to authorize their subscription or give account recovery instructions. For example:
The comparison table between email validation and verification:
Aspect | Email validation | Email verification |
Purpose | Ensures the email follows proper formatting rules | Confirms the email address actually exists and is active |
Process | Checks for structure, such as correct use of “@” and domain part | Sends a request to verify if the mailbox is real and receiving |
Use case | Useful for initial analysis of user input forms | Crucial for reducing bounces and validating active accounts |
Common method | Regular expressions or built-in PHP filters | DNS lookups for MX records and pinging the mail server |
Risk of false negatives | High (valid-looking emails can still be invalid) | Low (nearly confirms email usability if passed) |
The best time to use | On the client side, to quickly validate the format | On the server side, before sending important communications |
So, in some cases, validation may be sufficient. However, if you want to determine that the email address is associated with a real person, bet on verification.
Setting up PHP for email validation
Before diving into the code, go over the basic setup and some simple methods for validating emails.
What do you need before the start?
Before validating email addresses in PHP, you should have a few things in place:
- PHP environment – start with a solid environment. Check if your server is configured to handle PHP scripts properly.
- Server configuration – depending on whether you’re working locally or on a live server, it must handle outgoing requests. Especially if you plan on integrating email verification beyond basic validation.
- PHP libraries – make sure your PHP environment includes commonly used function filters like filter_var() to simplify validation tasks.
With these components ready, let’s move on to validating email addresses.
Meet basic email validation techniques
PHP offers a couple of straightforward techniques to determine if you’re dealing with valid emails. The two of them are:
- Filter_var()
Filter_var() is a built-in PHP function that filters and validates data, including email addresses. When used with the FILTER_VALIDATE_EMAIL filter, it checks if the given email address has a valid structure.
While it cares for the basic format, it does not fully guarantee that the domain is a fully qualified domain (FQDN) or that the address is valid in terms of actually existing and receiving emails.
- Regular expression
Regular expressions (regex) provide more granular control over email validation in PHP or other programming languages. They define specific patterns for what constitutes a valid address.
With regex, you can identify various components of an email, including:
- Special characters in the local part (before the “@” symbol)
- Domain formatting (like supporting internationalized domain names or limiting invalid characters)
While regular expressions offer more detailed and flexible control, they can get complex and may not catch all edge cases.
Validate email addresses in PHP: A step-by-step guide
Step 1: Validate email syntax with filter_var()
Use the filter_var() function to validate the basic format of an email address:
Step 2: Advanced validation with regular expressions
Use regex to create more customized validation rules:
Step 3: Domain validation
Now analyze if the domain in the email address is valid:
Step 4: MX record verification
To take domain validation a step further, you can verify if the domain has Mail Exchange (MX) records, which indicate that the domain can handle email traffic:
Step 5: Real-time email verification with PHP
For more advanced verification, consider using a third-party API service that provides real-time email validation.
Here’s an example using a hypothetical API:
Advanced techniques for email verification in PHP
If you’re dealing with a larger, more complex project – or simply want to ensure the utmost accuracy – explore some advanced techniques for verification and validation.
Below are a few ways to take your email verification to the next level.
- Integrate third-party services.
Why should you rely solely on basic PHP functions when you can incorporate powerful third-party email verification services into your application?
Tools like Bouncer offer comprehensive email verification API, toxicity check, deliverability kit, data enrichment, and many integrations!
Here’s a short example of how you can easily integrate a third-party email verification API into your PHP code:
- Handle disposable and role-based emails.
Disposable emails (think “temporary” or “one-time-use” email accounts) and role-based emails (like “admin@example.com” or “support@domain.com”) can clutter your database and reduce the quality of your contact list.
Two quick methods to flag disposable emails are:
- API. Many third-party email verification services offer built-in disposable email detection. If you already use such a service, check the API documentation for fields that flag disposable emails.
- Filters for role-based emails. You can handle role-based email addresses directly within PHP by creating a filter using a regular expression.
- Optimize PHP code for large-scale verification.
If you must verify hundreds or even thousands of email addresses, optimize your PHP code for bulk email analysis.
- Take the process in batch: divide your list into batches and process them together – it will reduce the load on your server and make it more efficient.
- Send asynchronous requests: for API-based verifications, you can improve efficiency by sending asynchronous API requests.
- Cache results: Cache and store the result in a database so you don’t have to repeat the procedure.
Common challenges and how to overcome them
When working with email validation in PHP, several challenges may arise that can impact your efficiency.
What are they?
01 Handling invalid emails
Invalid emails can occur due to typos, outdated information, or malicious intent. Thus, you can:
- implement robust error handling to handle these cases
- provide clear feedback to users and allow them to correct their email addresses
- discuss common issues and errors in email validation and how to handle them in PHP
02 Preventing false positives and negatives
It is not always possible to avoid false positives and negatives. Thus, you can use APIs for real-time verification or combine PHP’s basic validation methods with other techniques.
Then, you will reduce the chances of incorrectly validating or rejecting an email address.
03 Rate limiting and API usage
When dealing with large-scale email checking through third-party APIs, hitting rate limits can be a major concern.
Therefore, monitor API limits to prevent disruptions. Or simply use a tool with large limits, advanced APIs, and guaranteed speed and security.
Enjoy email address validation in PHP
By using PHP for this email validation, developers can filter out inaccurate email submissions early on and guarantee that only valid addresses are stored and used.
The best practice we can give you in 2025 is to always pair basic email validation with more advanced techniques, such as checking MX records. Also, regularly audit and optimize your validation logic to accommodate the latest standards and email formats.
If you need any help, use tools like Bouncer for more robust email verification.
Start our free trial and discover how easy it can be to validate and verify email addresses.
FAQs on PHP Email Validation and Verification
How do you validate an email in PHP?
Use filter_var() to check the email’s format and apply a regular expression for advanced validation to guarantee correct structure. As a result, emails conform to the proper syntax and avoid basic errors like missing characters.
How to email verification in PHP?
Verify emails by checking the domain’s MX records with checkdnsrr() or integrating third-party APIs for deeper validation. By doing so, you can see if the email’s domain is configured to accept messages.
How to check if email is real or not in PHP?
Use MX record checks with checkdnsrr() and real-time email verification services through API integration to confirm the email. Combining these approaches reduces the chances of invalid or non-existent emails entering your system.
How to check for existing email in PHP?
Query your database using SQL with PHP to see if the email is already present, ensuring no duplicate entries are allowed. This step helps maintain a clean, error-free database by avoiding redundancy.
How to validate a message in PHP?
Sanitize email content using filter_var() or htmlspecialchars() to guarantee the message body is free from harmful code or characters. Proper sanitization also helps defend against potential security risks like XSS attacks.
How to send confirmation mail in PHP?
Generate a unique confirmation link with a token, then send it to the user using PHP mail or PHPMailer for email authentication. Make sure to store the token in your database for later verification.
How to send email with PHP?
Send emails using PHP’s built-in mail() function or PHPMailer, which offers more robust and secure features for sending emails. Using PHPMailer also provides better handling for HTML-formatted messages and attachments.
How do I send an email verification?
Send an email with a verification link or token for user activation, typically after a user registers or submits their email address. Before activating an account, you ensure the user owns the email address.
How do I know if PHP mail is working?
Test mail() by sending a basic email to yourself, then check your mail logs or server’s error log for issues if it doesn’t work. This quick test determines if your mail setup is correctly configured.
How do I verify if an email exists?
Use MX records and SMTP validation via third-party services to validate if the email address exists and is able to receive mail. These methods are especially useful for reducing invalid email entries.
Which PHP function is used to validate an email address?
PHP’s filter_var() function is commonly used to validate email addresses by checking their format against defined standards. It’s a simple yet effective method for ensuring email validity.
How to verify email in PHP?
Validate with filter_var(), check MX records, and use third-party services for full email verification in PHP projects. Such a multi-layered approach improves both accuracy and reliability.
How do you check emails?
Parse email addresses with filter_var() or regular expressions to make sure they’re valid and correctly formatted before storing them. Always verify their domain and structure to avoid issues later on.
What is validation in PHP?
Validation in PHP is the process of verifying that user input (like emails or forms) meets specific criteria, preventing invalid or harmful data. Filter and sanitize inputs to maintain data integrity.