But how to verify an email address in Python?
Here’s the tutorial with the codes you can copy and paste to find out if you have an invalid email in your list.
⬇️ Short on time? See the quickest way at the end!
Method 1: using regular expression
A regular expression (also known as Python regex) is a powerful tool in any programmer’s toolbox for manipulating and validating an email address’s strings. The “re” module in Python provides full support for Perl-like regular expressions.
1. Import the re module
Install the re-module, which is built into Python, so there’s no need for additional installations. Simply add the following line at the beginning of your script.
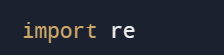
2. Define your regular expression for email validation
Next, define a regular expression pattern that matches a typical email address.
This pattern checks for:
- A sequence of alphanumeric characters, dots, underscores, percentages, and plus signs (local part of the email).
- The @ symbol.
- A domain name consisting of alphanumeric characters and dots.
- A top-level domain with a length of 2 to 7 alphabetic characters.
If an address is missing these details and valid characters, it’s an invalid email.
3. Develop an algorithm to validate email addresses
Write a helper function named “check” that uses the “re.fullmatch” command. It compares the entire email address string against your regex pattern to check any type of email address for validity.
4. Test the operation
Finally, test your script with different email strings to see it in action.
Be aware of these regular expression limitations
Regular expressions might struggle with:
- Complex local parts – The local part of an email address (before the @ symbol) can include a variety of characters and patterns that are difficult to capture accurately with a regex pattern.
- Domain validation – Regex patterns may also have difficulty verifying that the domain part of the email address is valid (after the @ symbol). In other words, this sort of validation just gives you a basic check of the format of the email address string, but nothing more.
- Internationalized email addresses – It might bring false results while handling domains in non-latin scripts or with special characters (which are increasingly common).
Method 2: validating from a text file
In real-world scenarios, you probably need to validate more emails than just a few. In this context, validating bulk emails from a file makes perfect sense.
1. Read emails from a text file
First, you need to read the email addresses from a text file. Assume you have a file named emails.txt, where each line contains an email address.
This script opens the file, reads each line (each email), and returns a list of emails, removing any extra whitespace at the same time.
2. Use the email validation function
Use re.search for email validation. The code will check each email read from the file to ensure email validity.
3. Validate emails from the list
It is now time to iterate through the email list and validate each one with the validate_email function.
The above code categorizes emails into valid and invalid lists and gives clearly output of which emails passed the validation test.
4. Testing the coding
To test the process, create a file named emails.txt and populate it with various email addresses (both true and false). Run the script to see how it categorizes each email.
Method 3: using email_validator
The email_validator library validates that a string adheres to the email format “[email protected],” e.g. while putting emails in login forms on the website. It provides user-friendly error alerts in cases where validation is unsuccessful.
Unlike regular expressions, email_validator performs thorough checks on an email address’s structure, domain validity, and overall integrity.
1. Install the email_validator library
Install the library using pip – the Python package installer. Run the following command in your terminal or command prompt.
2. Use the email_validator
Begin by importing the necessary functions from the email_validator library.
3. Create an algorithm
In your Python script, incorporate the library and use it to validate an email address.
4. Test it out
If valid, it returns the normal email form. If invalid, it provides a clear, human-readable error text.
Method 4: using re.match
Re.match methods use Python regex to check the appearance of email addresses. It examines the user’s name, and domain name – and makes sure the email contains all the necessary elements, like the @ symbol.
Please note: The algorithm spots lowercase letters, numbers, and special characters. But it can’t tell whether the email address exists or the domain works.
1. Add the re module
Start by importing the “re” again.
2. Define the email regex pattern
Define the pattern for a valid email address. A basic pattern includes control characters for the local part of the email, an @ symbol, and characters for domain names. Here’s a simple yet effective regex pattern for validating emails.
This pattern guarantees that the email address format starts with an alphanumeric character (including dots, underscores, and percentages), followed by the @ symbol, and ends with a domain name.
3. Validate the email
Finally, use the re.match() function to check if the email address matches the pattern.
It will return “Valid Email” if the input matches the pattern and an error otherwise.
4. Test it out
To thoroughly test the scripts and see whether they return false results, try them with a variety of emails.
The easier way to validate emails – an email verification service
Ever wished you could validate emails without needing to code? Wanted to find every invalid email address or temporary email in seconds?
Bouncer fills that gap.
It’s an easy-to-use tool that helps you clean up your email list with just a few clicks (literally a few!).
Moreover, it can check given email addresses hosted on various mail servers (including deep catchall Google Workspace and Office365 verification).
How to verify email addresses with this tool?
Step 1: Import your mailing list
Starting is easy. Sign up, create a user account, and just upload your email list to Bouncer.
It could be a CSV, TXT file from your computer, or a spreadsheet.
Alternative: if you’re looking for even more convenience, Bouncer can be integrated directly with the tools you already use so you can find out if something is a valid address straight from your registration form. This means you can validate email addresses in real-time and seamlessly blend Bouncer into your existing workflow.
Step 2: Start validating
With your list uploaded, hit the validate button. Bouncer quickly goes through your emails and spots the ones that might give you trouble.
There is no need to track the process and spend any time on it. It’s the Bouncer’s job to do this.
Once your list is verified, Bouncer sends you an email notification so you can immediately take the desired action to increase the value of your mailing campaigns.
It can spot disposable emails, spam traps and many other bad email types with high email validation accuracy.
➡️ See the email validation process in detail here: How to Check if an Email Address is Valid
Step 3: Get results in minutes
The beauty of Bouncer lies in its speed and efficiency (even 200,000 emails per hour!). This makes it
In no time, your email list is verified. You don’t have to wait long to get results.
This rapid turnaround is ideal for businesses and individuals who value time and efficiency.
What results will you get? The final outcome will be divided into four main categories – deliverable, risky, undeliverable, and unknown (usually very low at 0.3-3%).
That way, you can quickly delete unwanted emails that negatively affect your sender’s reputation.
In the end, let’s talk about money.
Bouncer gives you 100 credits for free – a nice way to test the waters. After that, you’ve got options: pay as you go, or pick a plan that suits your needs. For bulk email validation, Bouncer provides top accuracy and speed.
Bouncer helps your email reach people and bypass the spam filters. Not to mention that it’s way simpler than trying to master Python. For the time and headaches it saves, it’s a smart investment that pays back.
BONUS: You can also try out verifying email using JavaScript, and Excel. Read our guides and find out how.
Valid email addresses without using programming code
Without a doubt, it’s valuable to master Python for email validation. But it’s not the easiest path for everyone who wants to do a valid email check. The learning curve can be steep, and not everyone has the time or resources to dig into codes and scripts.
Think of Bouncer’s bulk email verification tool as the easy button to confirm that your emails are on point. Without any code. Give Bouncer a shot – it’s a smart move for hassle-free email verification.